SQLSTATE[42S01]: Base table or view already exists - Laravel Fix
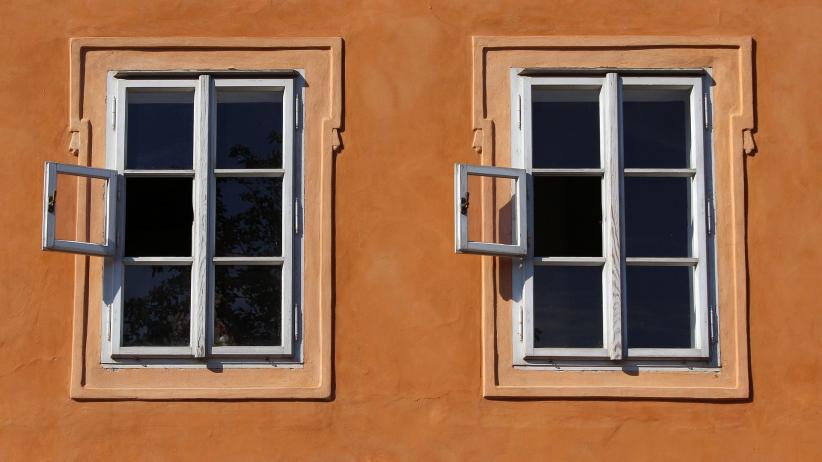
Base table or view already exists error occur when Laravel Migration is one of the most headache thing for newcomers when they start using migration. Base table or view is a kind of table in mysql. There are two items in database named table or view. If they exists already and you migrated, the problem arises.
Laravel 7 features require PHP 7.2.5
Migration Problem to reproduce
$ php artisan make:migration create_customers_table
There will be some migration file named 2014_10_12_000000_create_customers_table in database/migrations folder
Ok then, we create the migration as follows in public function up():
Schema::create('customers', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->string('password');
$table->timestamps();
});
However, You don't define anything in public function down(). THE PROBLEM ARISES.
Then You do migration refresh using
$php artisan migration:refresh
(or)
$php artisan migration:refresh --seed
You will now see the following error in second time refresh, if you don't run the migration table.
SQLSTATE[42S01]: Base table or view already exists in customers table .....
Cause of base table or view already exists
The main cause when first migration the up() function is called and your migration is worked well with customers table. However, when migration refresh is called, the down() function is called in customers migration file but there is no instruction for removal. So, the base table or view already exists and it still not removed.
So, when you migrate back or refresh migration, the old base table or view already exists and you will see the above error with SQLSTATE.
Solution to base table or view already exists
There is two solution.
1. Add Remove Instruction in public function down()
public function down() {
Schema::dropIfExists('customers');
}
2. Add Condition in public function up()
if (!Schema::hasTable('customers')) {
Schema::create('customers', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->string('password');
$table->timestamps();
});
}
I hope this thing will solve your problem. Thank you.
Read More about Laravel Migration in Official Site: https://laravel.com/docs/7.x/migrations